What is JavaScript
JavaScript (JS) is a lightweight, object-oriented programming language used for scripting web pages. It enables dynamic interactivity on websites and was introduced in 1995 with Netscape Navigator. Although its name suggests a connection with Java, JavaScript is entirely distinct from Java. Many modern web applications use JavaScript to provide interactivity without reloading the page. Databases like CouchDB and MongoDB also use it as a query language.
Features of JavaScript
- Supported by all popular web browsers
- Follows C language syntax, making it structured
- Weakly typed with implicit type casting
- Object-oriented with prototype-based inheritance
- Lightweight and interpreted
- Case-sensitive
- Supports multiple operating systems like Windows and macOS
- Provides control over web browsers
Application of JavaScript
JavaScript is used for:
- Client-side validation
- Dynamic drop-down menus
- Mathematical calculations
- Displaying pop-up windows and dialog boxes
- Displaying clocks
Checking Palindrome Number
JavaScript Code:
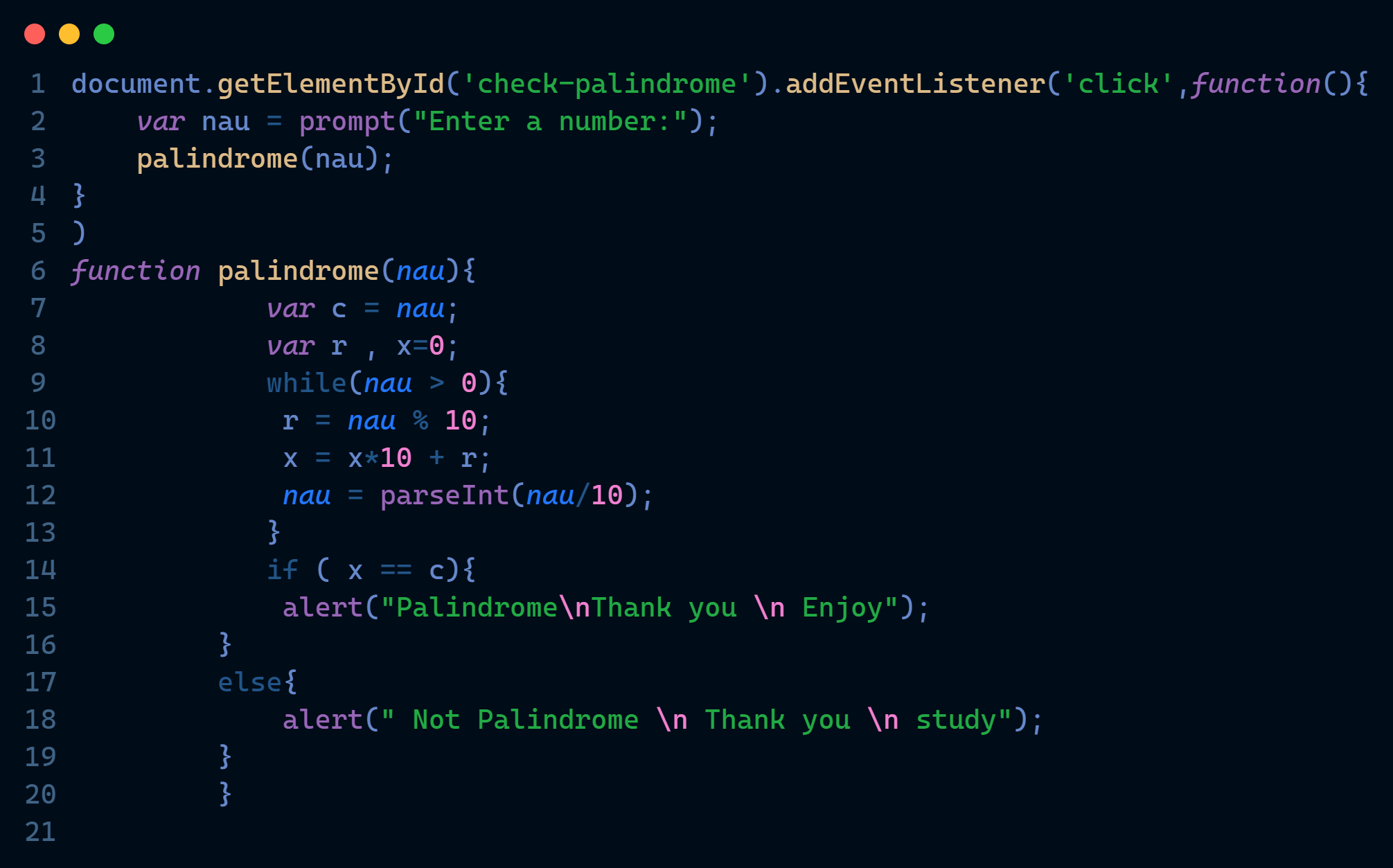
Output:
Checking Odd Even
JavaScript Code:
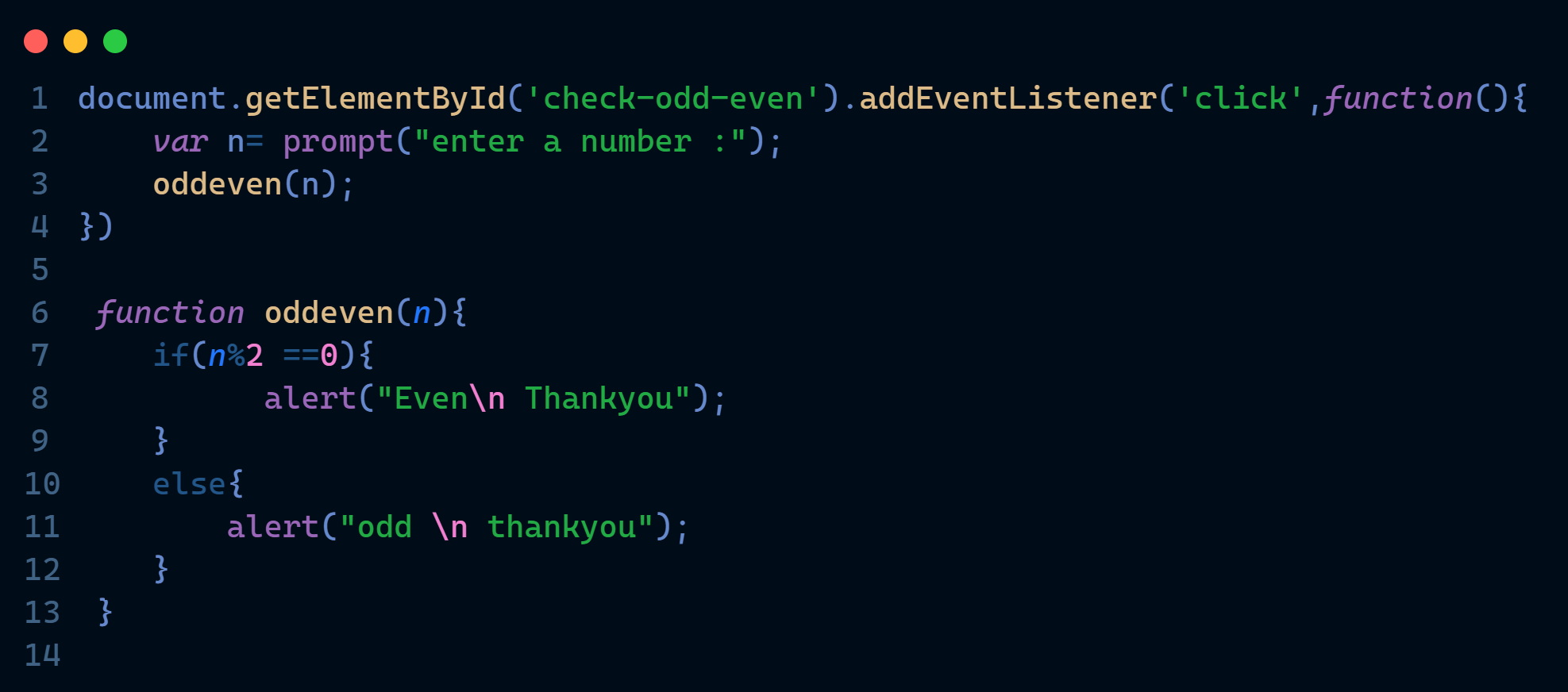
Output:
JavaScript Form Validation
Validating form inputs is crucial to ensure data accuracy. JavaScript provides client-side validation, which speeds up data processing compared to server-side validation. Developers often use JavaScript to validate fields like name, password, email, date, and mobile numbers.
JavaScript Form Validation Example
We validated fields for empty inputs, password length (minimum 6 digits), email, confirm password, and age (greater than 18).
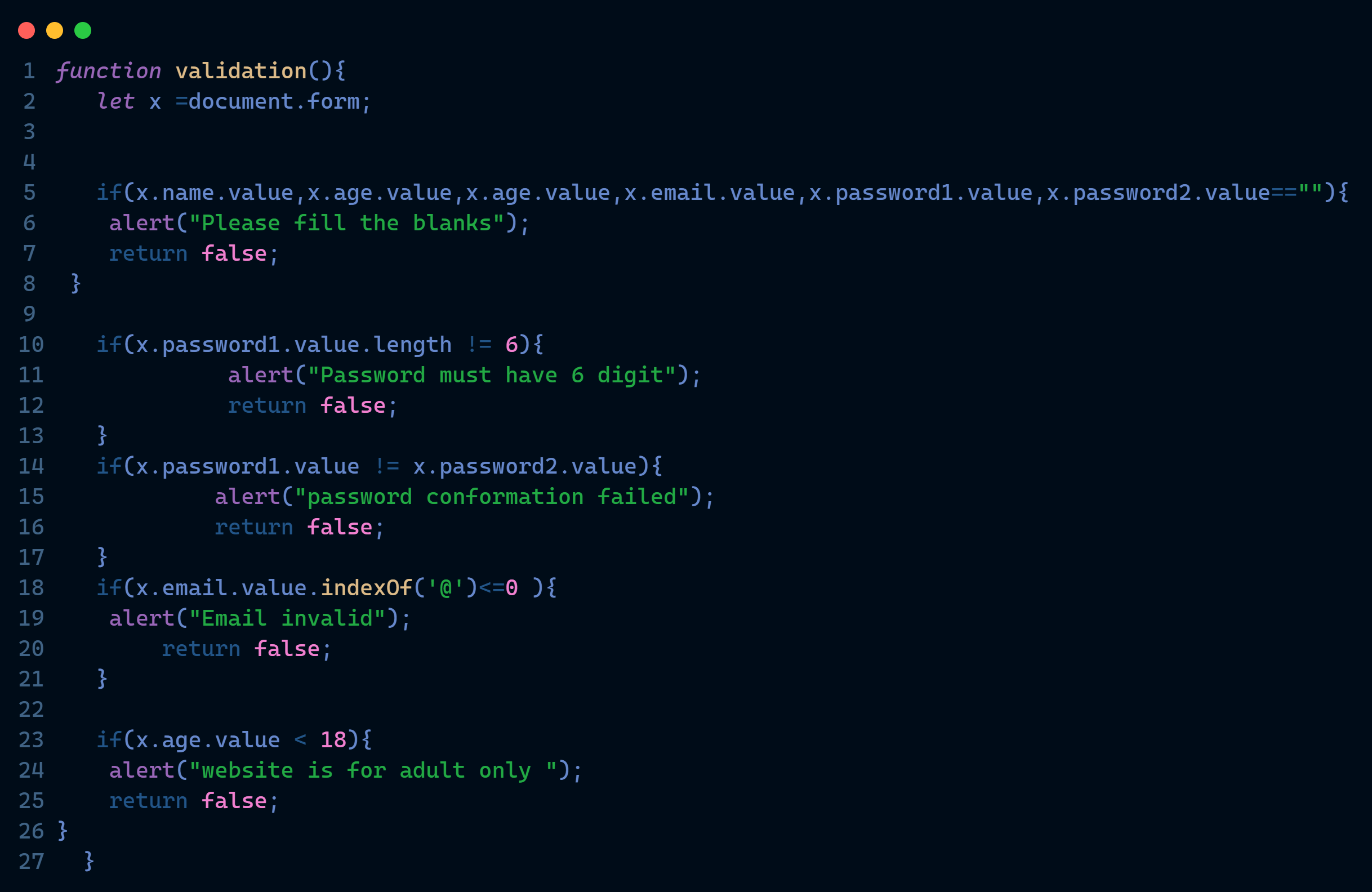
Event Handling in JavaScript
JavaScript provides an easy way to handle user events like clicks, form submissions, key presses, etc. These events allow developers to create highly interactive user interfaces.
Example of Event Handling:
document.getElementById("myButton").addEventListener("click", function() {
alert("Button clicked!");
});
Output:
JavaScript ES6 Features
JavaScript ES6 (also known as ECMAScript 2015) introduced several new features to make JavaScript more powerful and concise. Some of the key features include:
- Arrow functions for shorter syntax
- Let and Const for block-scoped variables
- Template literals for multi-line strings
- Destructuring assignment for unpacking values
- Promises for handling asynchronous operations
- Classes for object-oriented programming
Asynchronous JavaScript (Promises, Async/Await)
Handling asynchronous operations like API calls or file operations can be difficult using callbacks. JavaScript ES6 introduced Promises, which are objects that represent the eventual completion (or failure) of an asynchronous operation and its resulting value. Later, ES8 introduced async/await for a more synchronous-looking way to handle Promises.
Example with Promises:
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Data fetched!");
}, 2000);
});
}
fetchData().then(result => {
console.log(result);
});
Example with Async/Await:
async function getData() {
let result = await fetchData();
console.log(result);
}
getData();